- Published on
Getting Started with Spring Boot Framework
6 min read
- Authors
- Name
- Santosh Luitel
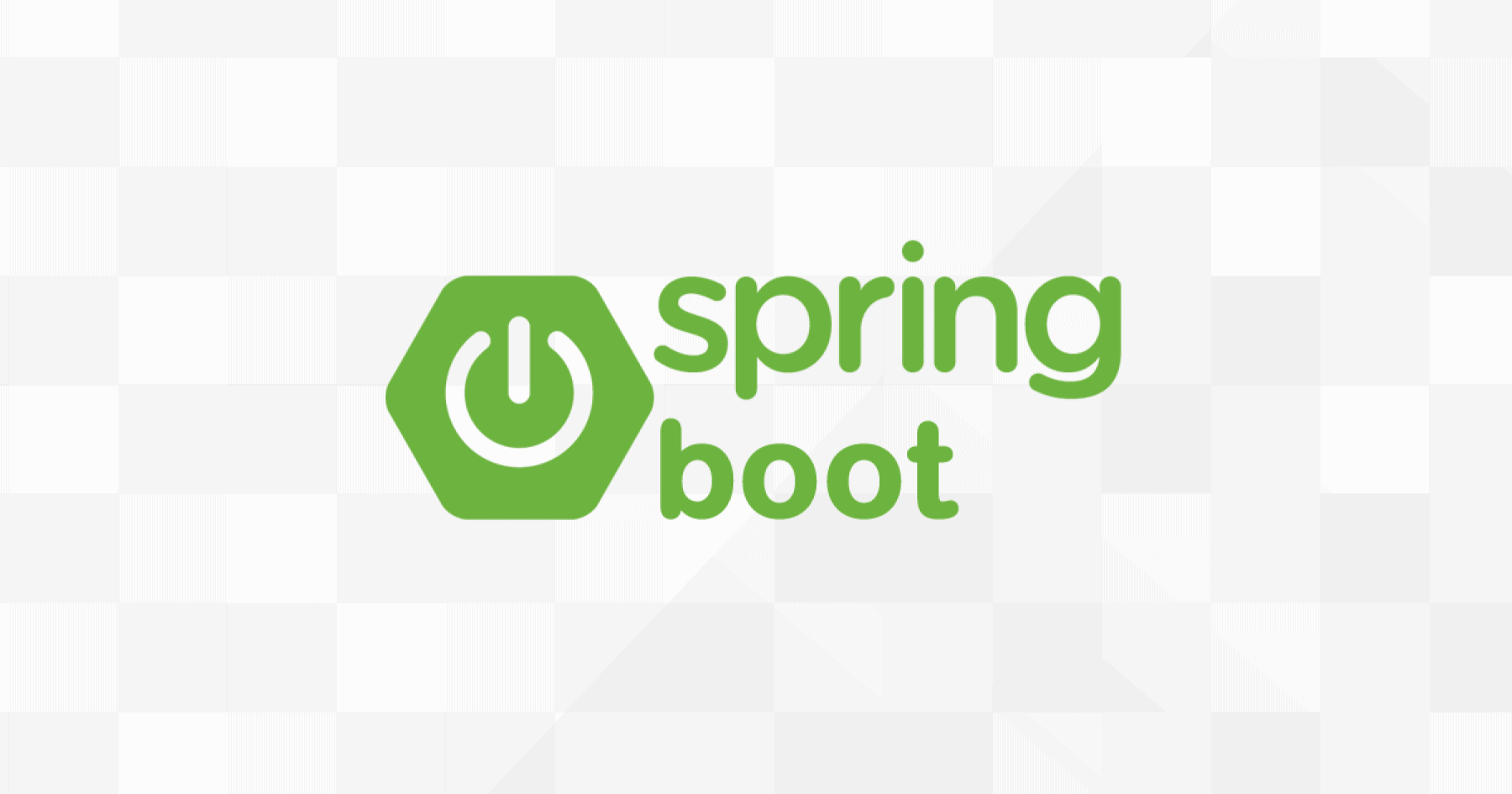
Table of Contents
In this article, we'll explore the heart of the Spring Boot framework—its auto‑configuration, starter dependencies, and embedded server—and build a simple REST microservice from scratch.
What is Spring Boot?
Spring Boot is a convention‑over‑configuration framework for Java that:
- 🔧 Auto‑configures beans based on the classpath
- 📦 Provides “starter” POMs for common features (Web, Data JPA, Security…)
- ⚡ Includes an embedded Tomcat/Jetty server—no external container needed
- 🔄 Bundles DevTools to restart on code changes
All this means you can focus on writing application logic instead of XML files and boilerplate.
Quickstart with start.spring.io
Generate a new project with:
- Project: Maven
- Language: Java 17+
- Dependencies: Spring Web, Spring Data JPA, H2 Database, DevTools
Or run:
curl https://start.spring.io/starter.tgz \
-d dependencies=web,data-jpa,h2,devtools \
-d javaVersion=17 \
-d name=demo \
-d packageName=com.example.demo \
| tar -xzvf -
Open DemoApplication.java
in your IDE—the entry point.
Project Structure
demo
├── src
│ └── main
│ ├── java/com/example/demo
│ │ ├── DemoApplication.java
│ │ ├── controller
│ │ │ └── BookController.java
│ │ ├── model
│ │ │ └── Book.java
│ │ ├── repository
│ │ │ └── BookRepository.java
│ │ └── service
│ │ └── BookService.java
│ └── resources
│ ├── application.properties
│ └── data.sql
└── pom.xml
Define the Entity
// src/main/java/com/example/demo/model/Book.java
package com.example.demo.model;
import jakarta.persistence.*;
@Entity
public class Book {
@Id @GeneratedValue
private Long id;
private String title;
private String author;
// getters & setters
}
Repository & Service
// BookRepository.java
package com.example.demo.repository;
import com.example.demo.model.Book;
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book, Long> {}
// BookService.java
package com.example.demo.service;
import com.example.demo.model.Book;
import com.example.demo.repository.BookRepository;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookService {
private final BookRepository repo;
public BookService(BookRepository repo) { this.repo = repo; }
public List<Book> findAll() { return repo.findAll(); }
public Book findById(Long id) { return repo.findById(id).orElse(null); }
public Book create(Book b) { return repo.save(b); }
public Book update(Long id, Book b) {
b.setId(id);
return repo.save(b);
}
public void delete(Long id) { repo.deleteById(id); }
}
REST Controller
// BookController.java
package com.example.demo.controller;
import com.example.demo.model.Book;
import com.example.demo.service.BookService;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.net.URI;
import java.util.List;
@RestController
@RequestMapping("/api/books")
public class BookController {
private final BookService service;
public BookController(BookService service) { this.service = service; }
@GetMapping
public List<Book> list() {
return service.findAll();
}
@GetMapping("/{id}")
public ResponseEntity<Book> get(@PathVariable Long id) {
Book b = service.findById(id);
return b != null ? ResponseEntity.ok(b) : ResponseEntity.notFound().build();
}
@PostMapping
public ResponseEntity<Book> create(@RequestBody Book b) {
Book saved = service.create(b);
return ResponseEntity.created(URI.create("/api/books/" + saved.getId())).body(saved);
}
@PutMapping("/{id}")
public ResponseEntity<Book> update(@PathVariable Long id, @RequestBody Book b) {
if (service.findById(id) == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(service.update(id, b));
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> delete(@PathVariable Long id) {
service.delete(id);
return ResponseEntity.noContent().build();
}
}
Configuration & Seed Data
# src/main/resources/application.properties
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:mem:testdb
spring.jpa.hibernate.ddl-auto=update
-- src/main/resources/data.sql
INSERT INTO BOOK (title, author) VALUES
('Clean Code', 'Robert C. Martin'),
('Spring in Action', 'Craig Walls');
Run & Test
./mvnw spring-boot:run
- H2 console →
http://localhost:8080/h2-console
- API endpoints →
http://localhost:8080/api/books
Conclusion
Spring Boot’s auto‑configuration and starter dependencies let you spin up production‑ready microservices in minutes—no boilerplate, no XML, just Java code. Next up, add security with Spring Security, containerize with Docker, or deploy to the cloud!